How to Convert a String to Int in Java
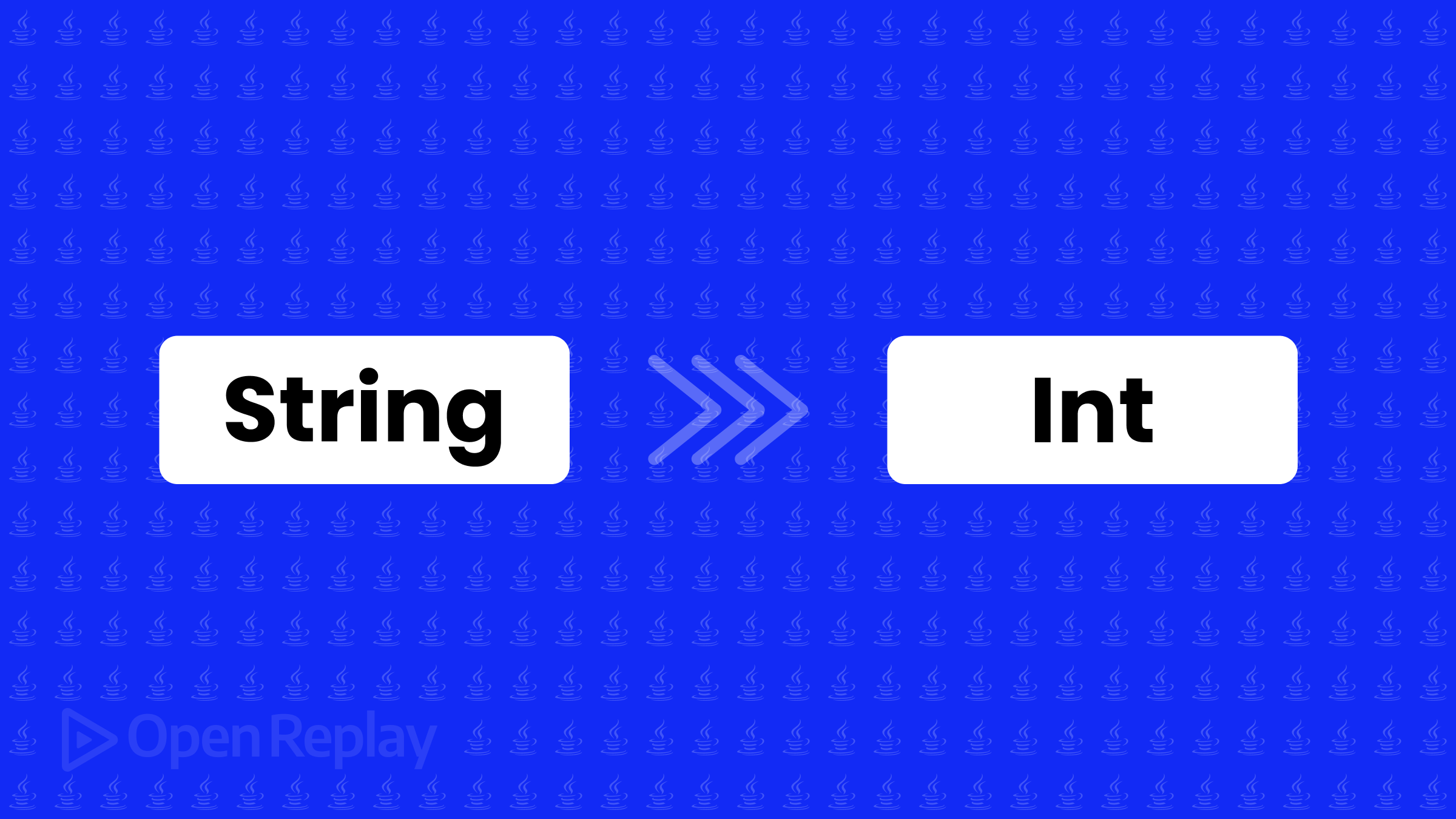
Converting a string to an integer is a common task in Java. Whether you’re processing user input or working with data from an external source, you’ll often need to handle this conversion safely and efficiently. This guide explores three simple methods to convert a string to an integer in Java.
Key Takeaways
- Use
Integer.parseInt()
for straightforward conversions. - Use
Integer.valueOf()
when you need anInteger
object instead of a primitiveint
. - Always handle potential exceptions, like
NumberFormatException
, to avoid crashes.
1. Using Integer.parseInt()
This is the most commonly used method to convert a string to a primitive int
:
String numberStr = ""42"";
int number = Integer.parseInt(numberStr);
System.out.println(""Converted number: "" + number);
Key Details
- Returns a primitive
int
. - Throws a
NumberFormatException
if the string cannot be converted (e.g., contains non-numeric characters).
When to Use This Method
Use it when you only need a primitive int
and are sure the input is a valid numeric string.
2. Using Integer.valueOf()
If you need an Integer
object instead of a primitive int
, use Integer.valueOf()
:
String numberStr = ""42"";
Integer number = Integer.valueOf(numberStr);
System.out.println(""Converted number: "" + number);
Key Details
- Returns an
Integer
object (wrapper class forint
). - Also throws a
NumberFormatException
for invalid strings.
When to Use This Method
This method is useful if you’re working with collections like ArrayList<Integer>
that require wrapper objects instead of primitives.
3. Handling Exceptions for Safe Conversion
Always handle exceptions when converting strings to integers to avoid runtime crashes:
String numberStr = ""42a""; // Invalid input
try {
int number = Integer.parseInt(numberStr);
System.out.println(""Converted number: "" + number);
} catch (NumberFormatException e) {
System.out.println(""Invalid number format: "" + numberStr);
}
Why Exception Handling Matters
- Ensures your application doesn’t crash on invalid input.
- Provides an opportunity to log errors or prompt users to input valid data.
FAQs
Both `Integer.parseInt()` and `Integer.valueOf()` will throw a `NumberFormatException`. Always check for null before attempting the conversion.
No. Both methods will throw a `NumberFormatException`. Use `Double.parseDouble()` or `Float.parseFloat()` for floating-point numbers, then cast to `int` if necessary.
A `NumberFormatException` will be thrown if the string represents a number outside the range of an `int` (-2,147,483,648 to 2,147,483,647).
Conclusion
Converting a string to an integer in Java is simple with methods like Integer.parseInt()
and Integer.valueOf()
. Always validate input and handle exceptions to ensure smooth execution in your applications.