GraphQL vs REST explained with code and use cases
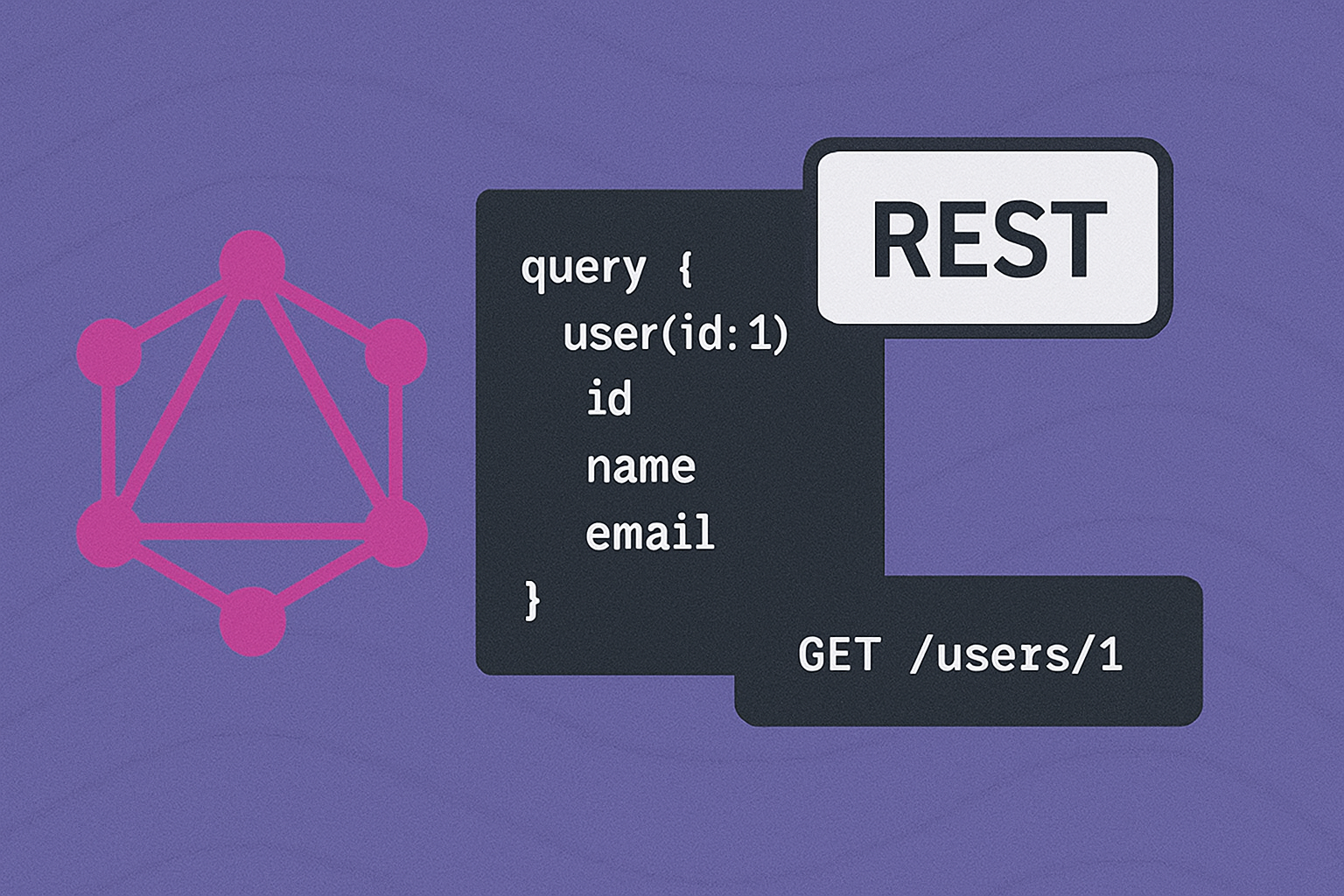
GraphQL and REST are two widely used approaches to API design. Each has strengths and trade-offs depending on how your application fetches, transforms, and updates data. This guide breaks down the core differences between GraphQL and REST, provides examples, and explains when to use one over the other.
Key Takeaways
- Understand the key differences between GraphQL and REST
- Learn when to use each based on application needs
- See real-world examples and performance trade-offs
What is REST?
REST (Representational State Transfer) is an architectural style that structures APIs around resources. Each resource is accessed through a unique URL using HTTP methods like GET, POST, PUT, and DELETE.
Example
GET /users
GET /users/1
GET /users/1/posts
Each endpoint returns a fixed data structure. REST is widely supported, cache-friendly, and easy to learn.
What is GraphQL?
GraphQL is a query language for APIs. Instead of multiple endpoints, there’s typically one /graphql
endpoint. Clients describe exactly what data they need, and the server responds with a matching structure.
Example
query {
user(id: 1) {
name
posts {
title
}
}
}
GraphQL responses return only what’s requested — no more, no less.
REST vs GraphQL: Feature Comparison
Feature REST GraphQL Data fetching Multiple endpoints Single flexible query Overfetching Common Avoided Underfetching Common Avoided Versioning URL or headers Schema evolution Caching Native HTTP-level Needs tools (Apollo, Relay) Learning curve Lower Higher File uploads Native Needs setup Tooling Mature and widespread Growing (Apollo, GraphiQL, etc.)
Real-world examples
REST
- Ideal for public APIs and CRUD apps
- Example: A blog API with endpoints like
/posts
,/comments
,/users
GraphQL
- Excellent for mobile and frontends with dynamic data needs
- Example: A dashboard that pulls a user, their projects, and activity logs in one request
Code comparison
REST: Fetch user and posts
GET /users/1
GET /users/1/posts
Client must combine responses manually.
GraphQL: Same in one query
query {
user(id: 1) {
name
posts {
title
}
}
}
Client gets all needed data in one response.
Performance and scaling
- REST benefits from browser and CDN caching. Great for static or read-heavy APIs.
- GraphQL minimizes payload size but needs safeguards (query depth limits, rate limiting).
- Overly complex GraphQL queries can impact backend performance if not managed.
When to use REST
- You have well-defined resources
- Your clients don’t change data needs often
- You want to leverage HTTP caching
When to use GraphQL
- Your frontend frequently changes or requests deep, nested data
- You want flexible queries from a single endpoint
- Your mobile apps or SPAs need lean payloads
Conclusion
Both REST and GraphQL are powerful. REST is simple, familiar, and works well for many use cases. GraphQL gives clients more control and efficiency, especially in complex apps. Choose based on your app’s data needs, frontend flexibility, and infrastructure.
FAQs
Not always. GraphQL is more flexible for complex data needs, but REST is simpler and better for public APIs with caching.
Yes. Many companies use REST internally and expose GraphQL as a unified API layer.
No. GraphQL is an alternative, not a replacement. Each fits different needs.