How to Autofocus using React Hooks
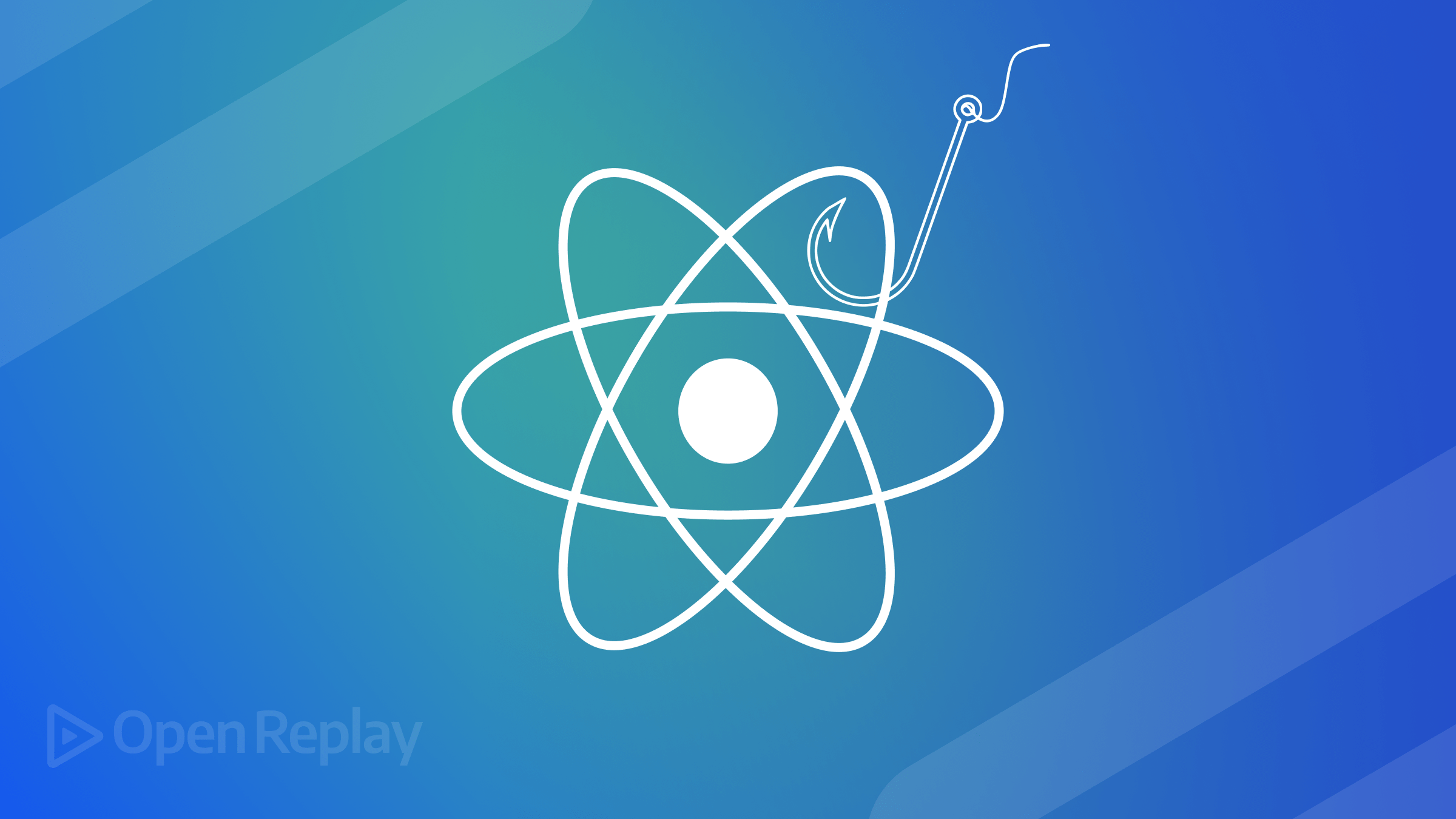
In web development, autofocus is a crucial aspect that improves the user experience by automatically focusing on input fields during page load or specific user interactions. This article will show you how to implement it with React by using hooks.
Discover how at OpenReplay.com.
Even though autofocus may seem like a minor detail, it can make workflows more efficient, improve user comfort, and most importantly, ensure that the important fields are always ready for data entry. Additionally, this may be used as an encouragement to speed up tasks such as filling out login forms and searching through websites since no one wants to spend time clicking on empty spaces before typing anything in such situations.
React is a famous JavaScript library that builds user interfaces. It serves as a declarative application state and behavior management tool. React Hooks’ emergence changed how developers interact with components and their logic by making them more concise and functional. Managing DOM elements such as input fields has become easier using functional components without manipulating DOM
directly through refs, effects, or other terminology-specific keywords associated with React’s declarative programming paradigm. A cleaner codebase is one of the benefits, along with increased predictability of application state and reduced debugging effort.
This tutorial will detail how to implement autofocus in React using hooks. We will begin by creating a simple react project and learning the essentials on which we base our hooks—useRef and useEffect among them. Afterward, we will show you how to create an input field that automatically gains focus when a component is mounted. In conclusion, some pitfalls are dealt with, and troubleshooting tips are provided to ensure that your implementation of autofocus works well throughout several situations and browsers.
Understanding the useRef
and useEffect
Hooks
The hooks useRef
and useEffect
should be comprehended well before being used in auto-focusing applications. These are efficient tools for accessing and changing parts of the model, enabling developers to create more versatile and interactive user interfaces.
A mutable reference to a DOM
element or any other value associated with a component throughout its lifetime can be created through the useRef hook, a React function. Essentially, this implies that when you call/refer to useRef
, what you get back is an object reference, which may be linked onto another material object—a DOM
element in this case—thus grabbing hold of all its characteristics and functions without making the component re-rendered again, thereby making it suitable for matters of controlling focus, among others, such as true auto-focus in an input field. Assigning this discounted version of the input element to useRef
makes it possible to alter it in any manner, including selecting text or programming to get a particular focus, etc.
On the other hand, the useEffect
hook performs side effects in functional components. Autocorrect uses this effect to run a piece of code focusing on a certain input area after the component has been rendered on DOM
. This function runs after the paint is done because useEffect
ensures that all elements in the DOM
are there and can be accessed. After employing useEffect
with useRef
, such an input element can be appropriately set to receive focus whenever its components are mounted, or particular dependencies change.
Let’s say you want your input field to be in focus as soon as the component renders for the first time. You can do this by creating a reference to it using useRef
and applying useEffect
to apply focus. Unlike open commands for any other element, open commands on selectors will be executed after the component has mounted. Also, by specifying dependencies, you can make useEffect
run whenever you want, allowing you to reapply focus according to different events or conditions, such as user interactions or changes in the application state.
The combination of useRef
and useEffect
can be a powerful tool for managing autofocus and other dynamic behaviors in React components. This enables developers to write clean, declarative code with the flexibility to perform imperative actions when necessary. Understanding how to use these hooks is crucial to building responsive and user-friendly React applications.
What we want to do
Let us set out to state our goal before looking at how we implement it. The goal is that, during the first render of a component, an input field should be automatically focused on. This makes things easier for end-users because they do not have to click the input beforehand when typing, thus enhancing the user experience. For that purpose, we will utilize React Hooks, such as useRef
, which allows referencing of the input element, and useEffect
, which ensures the immediate setting up of focus once a component has been rendered.
Setting Up the React Project
To implement autofocus in a React application through hooks, a React project must be set up first. Create React App
is an instrument used to establish a new React App that quickly provides an easy way to achieve this. The command you need to run starts with opening your terminal or command prompt:
npx create-react-app autofocus-example
The command builds a fresh folder, autofocus-example,
containing all essential files and settings needed for an elementary React app. After you finish setting things up, you can go to your project directory by using:
cd autofocus-example
Now let’s start the development server with:
npm start
Enter this command to start your fresh React application in the browser window. This will allow you to observe modifications during development.
Although a basic deployment covers all that is required for a simple React app, there may be additional packages that could interest you. For example, if you would rather go with TypeScript when it comes to checking data types, install all necessary files by using:
npm install typescript @types/node @types/react @types/react-dom @types/jest
Your project will have TypeScript added to it, together with type definitions for React and other necessary libraries. The use of TypeScript can aid in detecting possible mistakes early during programming and offer a more solid programming experience via static type checking.
Enhancing Autofocus in a Multi-Input Form
This part aims to create a comprehensive form with several input fields. The form will include fields for “First Name,” “Last Name”, and “Phone Number.” The idea is to implement key behaviors into these forms to enhance user experience.
First, we must ensure that when the page is loaded or the component is rendered, the focus goes straight to the “First Name” text box. This helps in forms where users are expected to start typing right away, making it easier for them not to click on the first field. This will be done through the useRef
and useEffect
hooks.
Next, we aim to establish dynamic interactions for other input fields. More specifically, we will add a feature that has to do with the input fields eliciting focal activities when they are hovered over. So, for example, when a user moves their mouse on top of “Last Name” or “Phone Number”, the respective fields become focused automatically. As such, this makes the form more intuitive and interactive since it reacts to all these mouse movements and actions without necessarily requiring more clicks.
Through these additions, we will have a form that not only attracts a user’s gaze during its first part but also improves user experience by moving from one field to another through other aspects of interaction that happen automatically and dynamically with users as they navigate through it. Now, let us look at how this can be done in code.
import React, { useRef, useEffect } from "react";
const AutofocusForm = () => {
// Create references for each input field
const firstNameRef = useRef(null);
const lastNameRef = useRef(null);
const phoneRef = useRef(null);
// Automatically focus on the first name field when the component mounts
useEffect(() => {
firstNameRef.current.focus();
}, []);
// Function to handle mouse over event and focus on the respective input
const handleMouseOver = (inputRef) => {
inputRef.current.focus();
};
return (
<form>
<div>
<label htmlFor="firstName">First Name:</label>
<input
ref={firstNameRef}
type="text"
id="firstName"
placeholder="Enter your first name"
onMouseOver={() => handleMouseOver(firstNameRef)}
/>
</div>
<div>
<label htmlFor="lastName">Last Name:</label>
<input
ref={lastNameRef}
type="text"
id="lastName"
placeholder="Enter your last name"
onMouseOver={() => handleMouseOver(lastNameRef)}
/>
</div>
<div>
<label htmlFor="phone">Phone Number:</label>
<input
ref={phoneRef}
type="text"
id="phone"
placeholder="Enter your phone number"
onMouseOver={() => handleMouseOver(phoneRef)}
/>
</div>
</form>
);
};
export default AutofocusForm;
Code explanation:
Then again, as part of making a website with a simple form having three fields named “First Name,” “Last Name,” and “Phone Number,” there is an introductory code to AutofocusForm
, which constitutes a React functional component whose main work is to focus on the first name input box each time the page or component loads and also when the user hovers his mouse over any of its two remaining input boxes.
To start with this component, we have firstNameRef
, lastNameRef
, and phoneRef
references made by the useRef
hook. When the component is rendered after that, these references will be attached to each single input field, hence allowing us to manipulate DOM
elements.
So as soon as the component mounts, the input field entitled “First Name” is automatically focused using the useEffect
hook (i.e., when the form is first rendered). This empty dependency array ([])
ensures that this effect only runs once when the component is mounted.
A helper function named handleMouseOver
has been created to enable the handling of mouseover events. It takes an input field as its parameter and calls its focus()
method if the user hovers over the respective input. This action is assigned to each input field using the onMouseOver
attribute. That means, whenever the mouse cursor moves above any of these input boxes, it will be activated to focus automatically.
In the return
statement, there are three labeled input fields. Each field’s ref
attribute refers to its reference (created via user) and has an onMouseOver
event that allows a dynamic focus based on mouse hover. The effect is that when interacting with this form, both component loading and mouse movement activate the inputs, which improves the overall user experience.
Expected output:
The AutofocusForm
displays a form to the user with three input fields called “first name,” “last name,” and “phone number.” When the page loads, the “First Name” field will have an auto-focus feature, which means that the blinking cursor will appear inside it and allow the person to continue typing. This action is controlled by the useEffect
hook, as it ensures that focus is set on the “First Name” input when the form appears on the screen.
Like “Last Name” or “Phone Number,” hovering over other fields will automatically cause focus to be directed towards an input field. By moving your cursor on the last name box, for example, it will be positioned so one can begin typing promptly without even clicking on it first. The same is the case with the phone number field. Such interactivity is achievable because whenever any mouse moves across one of these input areas, the handleMouseOver
function ensures it is focused.
Therefore, the general user encounter will be seamless and effortless. Furthermore, it is easy for users to enter their first names by loading forms, as attention is directed towards each area as soon as they hover over any other field. Thereby, no extra clicking is needed, increasing interactivity and making it much easier for users to fill out forms quickly.
Common Pitfalls and Troubleshooting
Developers may face common pitfalls and challenges when implementing autofocus using react hooks. In different scenarios, the reliability and consistency of autofocus may be compromised by delayed rendering of the components and browser-specific eccentricities, among others. It is important to understand these problems and know how to troubleshoot them to have a smooth user experience.
Delayed focus is one of the most common problems developers encounter concerning autofocus. It occurs when the input field doesn’t get immediate attention after component mounting. Various factors can lead to this problem, including input not being fully rendered by the time focus function or other asynchronous behaviors that interfere with autofocus logic. Delayed focus can be best handled by ensuring the input element is available and ready before applying focus. Place autofocus logic inside the useEffect hook with an empty dependency array so that it runs only once immediately after mounting. Alternatively, if it’s conditional, derived from other parts, or uses asynchronous data, developers might consider using additional hooks like useLayoutEffect
or apply focus in response to state change.
Another thing that frequently pains us is maintaining uniformity across the various open doors to the web. Autofocus functionality may be inconsistent among different browsers since they have differences in how to focus events and render updates work. Some browsers may impose a delay on focus across any text fields that are located outside the screen or that are not being dealt with at that particular moment. However, to eliminate these inconsistencies, it would be better to test this feature on all popular platforms to detect discrepancies. In instances where specific browser behaviors cause problems, developers can use conditional logic to modify how auto-focus is handled depending on the browser they identify or could apply CSS styles to each respective browser, thus achieving visual similarity.
Developers should also look into the possible implications of autofocus for accessibility purposes. This feature might enhance the user experience by directing attention toward particular input fields; if carelessly used, it has the potential to disturb keyboard navigation and even screen reader functionality. For instance, screen reader users can get confused when an input field gets automatically focused without their own volition; keyboard shortcut-dependent users can be distracted. Hence, to make it easier for everybody, including those with disabilities, to access web pages, developers must be restricted when using autofocus and concentrate on how to visually notify people concerning focused elements respectively. Developers have other choices, too, like managing focus through establishing focus.
Managing autofocus for complex applications may include dealing with state transitions and renders that influence when and where the focus needs to be applied. For example, a form containing dynamically inputted fields would require autofocusing to be set upon new ones or even changed in line with form validation errors. Therefore, developers should use the useEffect
hook with suitable dependencies to trigger focus changes under some circumstances only. This helps to ensure that focusing behavior is always predictable and responds to what users do or changes in the app’s state, thus making it better for all its users.
Conclusion
To sum up, the experience of an individual using an input field can be made greater using hooks such as useRef
and useEffect
for autofocus implementation in React. With a clear understanding of these hooks and meticulous control over focus behavior, the developers can create adaptive, user-friendly interfaces. It is important to note the common pitfalls, such as delayed focusing, browser compatibility issues, and accessibility concerns. Providing better practices and testing widely helps to have a consistent autofocus across different scenarios, which ensures smooth and intuitive handling for all.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.