Modern Alternatives to javascript:location.reload(true): How to Force a Page Reload in JavaScript
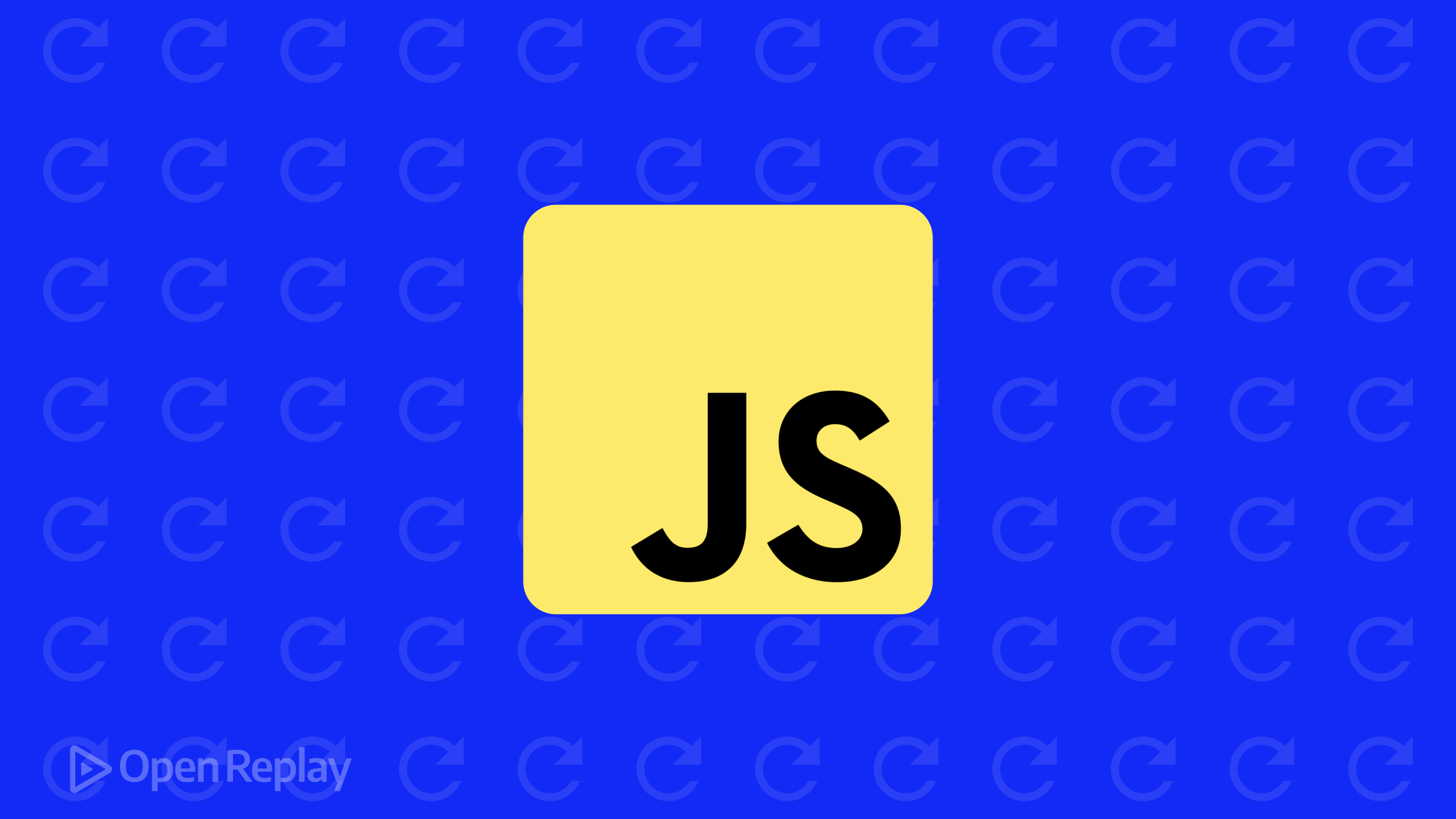
Are you still using location.reload(true)
in JavaScript to force a page reload? While this method works in some browsers, the forceGet
parameter is deprecated and not part of web standards. In this concise guide, we’ll explore modern, cross-browser alternatives to javascript:location.reload(true)
for refreshing a web page and bypassing the cache.
Key Takeaways
location.reload(true)
is deprecated. Use alternative, cross-browser methods- Append a unique query parameter to force a reload and bypass cache
window.location.reload()
without arguments is the standard reload method- Avoid page reloads when possible for better performance and UX
Understanding location.reload()
The location.reload()
method reloads the current URL, similar to the browser’s Refresh button. It has an optional forceGet
parameter (default false
) that, when true
, bypasses the cache and reloads the page from the server. However, this parameter is non-standard and only supported in Firefox.
Why location.reload(true)
is Deprecated
The forceGet
flag in location.reload(true)
is deprecated because:
- It’s not part of the HTML specification
- It’s only supported in Firefox, leading to cross-browser inconsistencies
- There are more reliable, standardized ways to force a reload
Modern Methods to Force a Page Reload
Here are current, cross-browser techniques to reload a page without using location.reload(true)
:
1. Append a Timestamp Query Parameter
Append a unique query parameter to the URL to trick the browser into thinking it’s a new page. A timestamp works well:
window.location.href = window.location.href + '?timestamp=' + new Date().getTime()
2. Use window.location.reload()
without forceGet
Simply call reload()
without any arguments. While this doesn’t guarantee a cache bypass, it’s the standard, cross-browser way:
window.location.reload()
3. Use window.location.href
Assignment
Assign window.location.href
to itself to reload:
window.location.href = window.location.href
However, this adds a new history entry. To avoid that, use window.location.replace()
:
window.location.replace(window.location.href)
Best Practices for Page Reloads
- Avoid reloading if possible. Update content dynamically with AJAX or use a SPA framework
- If you must reload, use standard methods like appending a query parameter
- Consider performance and UX implications of reloading
- Watch out for infinite reload loops
FAQs
Conclusion
By understanding why javascript:location.reload(true)
is deprecated and using modern alternatives like appending query parameters or window.location.reload()
, you can ensure your JavaScript code is standards-compliant and works consistently across browsers.