How to Generate PDFs in a React App
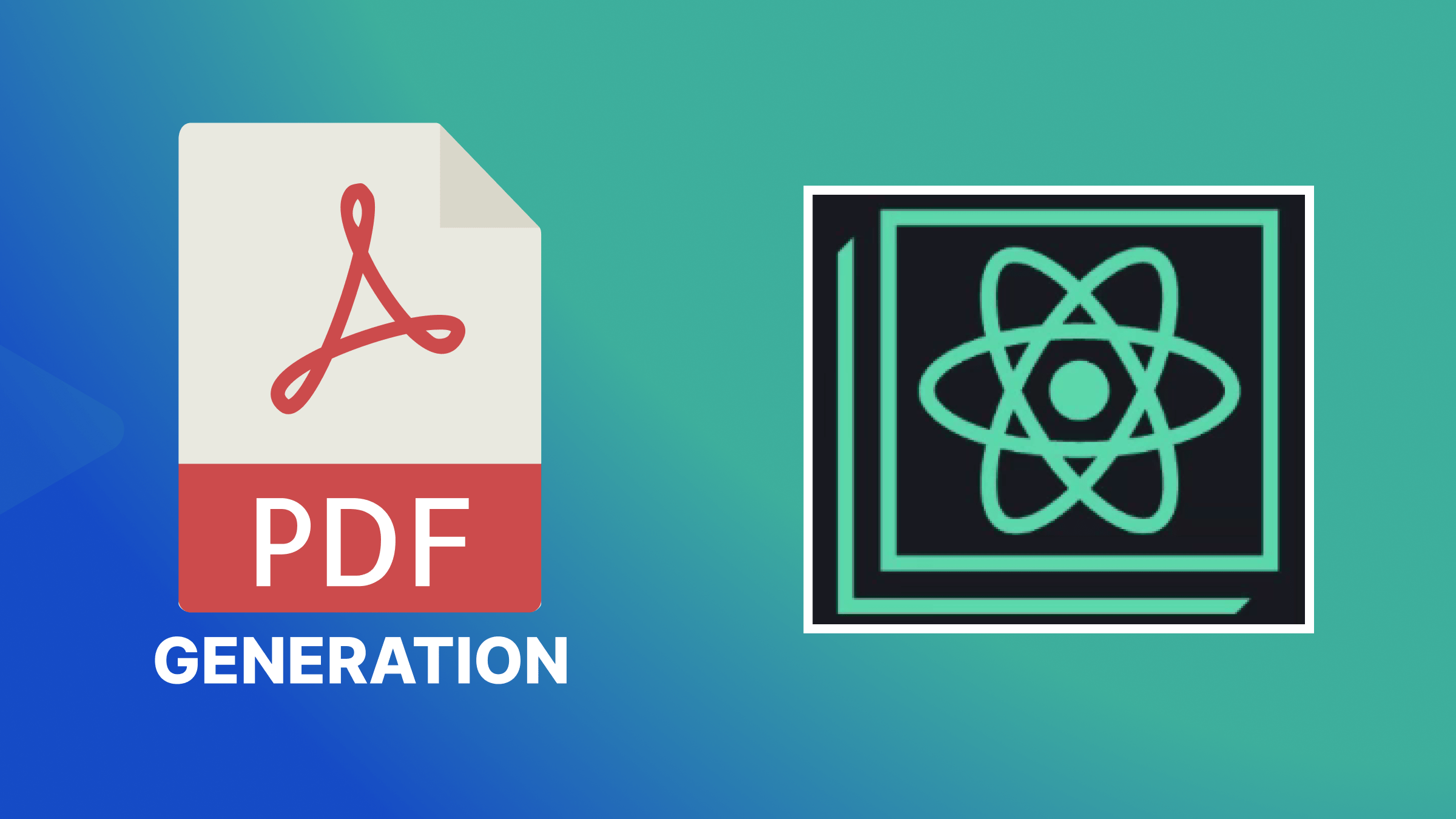
Incorporating PDF generation functionality in your React app provides a powerful tool for improving your user experience. Whether creating reports, generating invoices, or producing printable documents, this feature amplifies a developer’s ability to drive engagement and conservation to their app. This article will show you how to do exactly that, adding PDF generation to your React code.
Discover how at OpenReplay.com.
In today’s digital age, the ability to generate PDF documents dynamically has become increasingly valuable for web developers looking to enhance the functionality of their web apps. With React being one of the most popular frontend frameworks, It allows developers to seamlessly integrate these features into their React apps.
PDF generation offers users the convenience of exporting data and content in an easily shareable and printable format. This article will serve as a comprehensive tool for adding this feature to your code.
Use Cases of PDF Generation Feature
Here are some of the diverse applications of this feature in a React app:
- Receipt generation: This feature can be used to dynamically create and deliver digital receipts to customers after purchasing. This provides customers with the convenience of recording their purchases and facilitating returns. The digital receipt can be shared and downloaded for offline usage.
- Document generation: This feature can be used to create different types of documents such as certificates, contracts, and proposals. It can be used by developers to dynamically populate document templates with user-provided data and generate personalized documents tailored to your specific user needs.
- Document conversion: React apps can use the PDF generation feature to convert reports from various formats such as CSV, Excel, JPG, or GIF into PDF documents that are easily shareable and printable. These features streamline the entire process, whether it’s converting images, financial statements, or data analysis results.
- Educational resources: Educational websites built with React can use the PDF generation feature to automate the provision of downloadable resources like course materials, assignments, and lesson plans. The feature enhances their use for offline reading, printing, and sharing.
From the use cases above, it’s evident that these features offer a multitude of benefits to businesses across various industries for enhancing productivity and efficiency.
Using React-pdf for PDF Generation
React-pdf is a library packed with features that simplify generating PDFs in React apps. From converting different content types to advanced customization, this library provides web developers with a robust set of tools to seamlessly generate high-quality PDF documents directly within their React app.
Here are the features that make this library the go-to solution for anything PDF generation in React apps:
- Rendering options: React-pdf offers flexible rendering options, allowing generated PDF to be seamlessly displayed directly within the web browser or saved as a downloadable file.
- Browser compatibility: With robust support for all modern browsers, including Chrome, edge, safari, and Firefox, React-pdf ensures consistent performance across a wide range of environments.
- Component library: React-pdf boasts a rich collection of components that supports the rendering of various content in PDF format. It has various specialized components for text, images, graphics, pages, etc.
- Customization options: This library provides a plethora of component-specific props that customize the structure of PDF documents. It also supports the integration of CSS styling and font modules to enhance the visual aesthetics of the document.
React-pdf offers developers a seamless and efficient solution for PDF generation in React apps. It has versatile rendering options, comprehensive browser compatibility, and flexible customization capabilities. These features all together empower developers to enhance the user experience and functionality in their React apps.
Getting started
Before getting started on this project, there are some prerequisites you need to have. They include:
- A computer.
- A text editor.
- having React >= 16.8 installed.
- A basic knowledge of writing React code.
You’ll need to install the React-pdf library when all the prerequisites are settled. This can be done with either npm
, yarn
, or pnpm
.
First, open your terminal and navigate to the root directory of your React project.
Next, you run one of the following commands:
//npm
npm install @react-pdf/renderer
//yarn
yarn install @react-pdf/renderer
//pnpm
pnpm install @react-pdf/renderer
Once the installation is complete, you need to import into your code:
import { Document, Page, View, Text, StyleSheet } from '@react-pdf/renderer';
In the code above, we imported the Document
, Page
, View
, Text
, and StyleSheet
components from our package.
Document
represents the pdf document. Page
represents a single page in the document. View
represents a content block for wrapping the sections of the document UI. Text
allows the displaying of textual content. StyleSheet
is used for applying CSS styling on the document.
Here’s how you can implement a basic PDF generation component using React-pdf:
//Pdf.js
import { Document, Page, View, Text, StyleSheet } from '@react-pdf/renderer';
function PDF(){
let styling = StyleSheet.create({
page: {
backgroundColor: 'Khaki'
},
section: {
margin: 20,
fontSize: '16pt'
}
});
return (
<Document>
<Page style={styling.page}>
<View style={styling.section}>
<Text>Hello World</Text>
</View>
<View style={styling.section}>
<Text>This is a demo</Text>
</View>
</Page>
</Document>
)
}
export default PDF;
You can use the PDFViewer
and PDFDownloadLink
components to render the generated PDF and display a download link on the web browser.
//app.js
import {PDFViewer, PDFDownloadLink} from '@react-pdf/renderer';
import PDF from './Pdf.js';
function App(){
return (
<div className="app">
<PDFViewer>
<PDF/>
</PDFViewer>
<PDFDownloadLink document={<PDF />} fileName="demo.pdf">
{({ blob, url, loading, error }) =>
loading ? "Loading document..." : "click to download!"
}
</PDFDownloadLink>
</div>
)
}
export default App;
From the code above, the PDFDownloadLink
component has 2 props: document
and fileName
. The document
prop stores the PDF component that’ll be downloaded. the fileName
prop sets the document’s filename.
If everything goes well, a PDF document and a download link will be rendered in your browser.
Components
A robust set of components in React-pdf streamlines the PDF generation process. Some of them include Document
, Page
, View
, Image
, Text
, Link
, and PDFViewer
.
Document
This is a compulsory component that represents the pdf document. There can only be one document component and it must always be at the top. Its direct children must always be the Page
type. This component has various props that specify the metadata of the document. They include:
title
: This sets the document title on the metadata.author
: This specifies the author’s name.language
: This specifies the document’s default language.keywords
: This specifies the set of keywords associated with the document.pdfVersion
: This specifies the version of the generated PDF.pageMode
: This specifies how the document should be displayed when viewed in the browser. Its value can beuseNone
,useOutlines
,useThumbs
,fullscreen
,useOC
,useAttachments
.pageLayout
: This specifies the layout of the document. It’s valued can besinglePage
,oneColumn
,twoColumnLeft
,twoColumnRight
,twoPageLeft
,twoPageRight
.
Page
This represents a single page in the pdf document. A document can have as many pages as you want. It must also be a direct descendant of the document component. Here are some of the props that can be applied to this element:
size
: This specifies the page size. Its default value isA4
. It can have one of the following values:A0
toA10
,B0
toB10
,C0
toC10
,RA0
toRA4
,SRA0
toSRA4
,EXECUTIVE
,FOLIO
,LEGAL
,LETTER
, andTABLOID
.orientation
: This specifies the page orientation. Its default value isportrait
. It can also belandscape
.wrap
: The specifies the page wrapping for the page. Its default value istrue
; whenever there’s not enough space for content to be entirely rendered on a page, the remaining will be passed to a new page.False
means that the entire content will be rendered on a new page if the space isn’t enough for it.style
: This prop helps in defining the CSS styling for the page.id
: This specifies the page’s ID. It’s particularly useful for document navigation. When you click on a link that points to the id of a page, the view will be moved to the page.dpi
: This specifies the custom dpi for the page content. The default value is 72.
Other Components
The View
component represents a content block within a page. It acts as a direct parent for all the content that React-pdf supports. The content includes text, link, image, canvas, and SVG. The props are similar to that of the Page
component. They include wrap
, style
, id
, etc.
The Image
component represents the JPG or PNG images to be added to the PDF. Its core prop is the src
. This prop specifies the source of the image. Its value can be a string representing an image URL, filesystem path, or a base64 encoded image string. Other props are style
, cache
, etc.
The Text
component is used for displaying text. Its props include wrap
, style
, id
, etc.
The Link
component displays hyperlinks, which are particularly useful for document navigation. For it to work, it must have a src
prop whose value can be a URL or the ID of another page( or content) in the document.
Additional props are wrap
, style
, etc.
The PDFViewer
component represents an iframe PDF viewer. Its props can be style, className
, width
, height
, etc.
Styling the Document
React-pdf supports the use of CSS to style the PDF.
You can pass the styling as an object to the style
prop:
function PDF(){
return (
<Document>
<Page style={{margin:15,border:'1px solid black'}}>
<View style={{margin:15}}>
<Text style={{display:'block', padding: 10,color: 'blue',fontSize: '17pt', fontWeight: 900}}>Central bank of Nigeria, Monetary policy department</Text>
<Text style={{display:'block',padding:10,backgroundColor: 'silver', fontSize: '14pt', color:'blue', borser: '1px solid black'}}>Monetary policy series</Text>
</View>
<View style={{margin: 15}}>
<Text style={{display:'block',padding:10,backgroundColor:'limegreen', padding:10, color:'red' }}>WHAT IS MONETARY POLICY?</Text>
</View>
</Page>
</Document>
)
}
export default PDF;
You can also use the styleSheet.create()
method from the Stylesheet
module.
import { Document, Page, View, Text, StyleSheet } from '@react-pdf/renderer';
function PDF(){
let styling = StyleSheet.create({
page: {
margin: 15,
border: '1px solid black'
},
section: {
margin: 15
},
text1: {
display: 'block',
padding:10,
color: 'blue',
fontSize: '17pt',
fontWeight: 900
}
text2: {
display'block',
padding: 10,
backgroundColor: 'silver',
fontSize: '14pt',
color: 'blue',
border: '1px solid black'
},
title: {
display: 'block',
backgroundColor: 'limegreen',
padding: 10,
color: 'red'
}
});
return (
<Document>
<Page style={styling.page}>
<View style={styling.section}>
<Text style={styling.text1}> Central bank of Nigeria, Monetary policy department</Text>
<Text style={styling.text2}>Monetary policy series</Text>
</View>
<View style={styling.section}>
<Text>WHAT IS MONETARY POLICY?</Text>
</View>
</Page>
</Document>
)
}
export default PDF;
Regardless of the styling method you choose, this will render a style PDF document in your web browser:
There is support for the following CSS properties:
- Flexbox:
flexDirection
,alignContent
,flex
, etc. - Border:
borderStyle
,borderColor
,borderWidth
, etc. - Margin:
marginTop
,marginRight
,marginLeft
, etc. - Padding:
paddingTop
,paddingBottom
,paddingRight
, etc. - Color:
color
,opacity
,backgroundColor
. - Text:
fontSize
,textDecoration
,fontWeight
, etc. - Dimension:
width
,height
, etc. - Layout:
display
,position
,top
, etc.
Adding Fonts
React-pdf also supports the use of fonts. It has a Font
module that allows you to add multiple fonts to your document.
Here’s how it works:
import {Document, Page, View, Text, Font, StyleSheet} from '@react-pdf/renderer';
let sourcePoppins = 'https://fonts.googleapis.com/css2?family=Poppins:ital,wght@0,100;0,200;0,300;0,400;0,500;0,600;0,700;0,800;0,900;1,100;1,200;1,300;1,400;1,500;1,600;1,700;1,800;1,900&display=swap';
let sourceRoboto = 'https://fonts.googleapis.com/css2?family=Roboto:ital,wght@0,100;0,300;0,400;0,500;0,700;0,900;1,100;1,300;1,400;1,500;1,700;1,900&display=swap';
Font.register({family: 'Roboto', src: sourceRoboto })
Font.register({family: 'Poppins', src: sourcePoppins })
function PDF(){
let styling = StyleSheet.create({
section1: {
fontFamily: 'Roboto',
fontWeight: 700
},
text1: {
fontFamily: 'Poppins',
fontWeight: 700
},
text2: {
fontFamily: 'Poppins',
fontWeight: 400
}
});
return (
<Document>
<Page>
<View style={styling.section1}>
<Text>OpenReplay</Text>
</View>
<View>
<Text style={styling.text1}>Session replay you can self-host</Text>
<Text styling={styling text2}>All you need yo observe, measure, and iterate on your product</Text>
</View>
</Page>
</Document
);
export default PDF;
Result:
From the code above, we imported the Font
module alongside other components, then we used the Font.register()
method to register 2 fonts: . The Font.register()
method has an object argument with 2 compulsory properties: family
and source
. The family
property specifies the name of the font while source
sets the URL of the font.
Best Practices
Here are some of the things you must do to ensure that your PDF generation is seamless:
- Ensure that your code is correctly written to prevent errors
- Use memorization techniques to prevent unnecessary rendering of your component
- Optimize your PDF file size by compressing images and avoiding unnecessary elements.
- Be sure to follow up-to-date security measures when generating a pdf with sensitive information.
- If your PDF is large or has more than 30 pages, consider using web workers to generate them.
Conclusion
React-pdf emerges as a powerful tool for PDF generation in React apps. its comprehensive feature set empowers developers to streamline the document creation process and improve user experience.
Readers are encouraged to utilize this amazing tool in their projects. Whether generating reports, receipts, or any other type of document, React-pdf equips you with the tools to elevate your application to new heights.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.